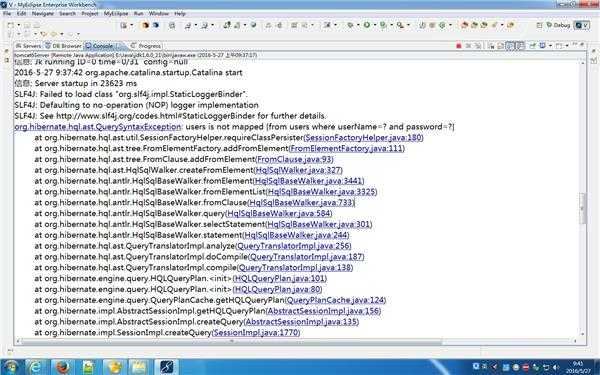
各位高手,帮本人看看问题出在哪里
UserInf
package pojo;
import java.sql.Timestamp;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Table;
/**
* Users entity. @author MyEclipse Persistence Tools
*/
@Entity
@Table(name=”users”
,catalog=”ehome”
)
public class Users implements java.io.Serializable {
// Fields
private Integer userId;
private String userName;
private String password;
private String contactName;
private String mobilePhone;
private String officePhone;
private String address;
private String email;
private String status;
private Timestamp createDate;
// Constructors
/** default constructor */
public Users() {
}
/** minimal constructor */
public Users(String userName, String password, String contactName, String mobilePhone, String address, String status, Timestamp createDate) {
this.userName = userName;
this.password = password;
this.contactName = contactName;
this.mobilePhone = mobilePhone;
this.address = address;
this.status = status;
this.createDate = createDate;
}
/** full constructor */
public Users(String userName, String password, String contactName, String mobilePhone, String officePhone, String address, String email, String status, Timestamp createDate) {
this.userName = userName;
this.password = password;
this.contactName = contactName;
this.mobilePhone = mobilePhone;
this.officePhone = officePhone;
this.address = address;
this.email = email;
this.status = status;
this.createDate = createDate;
}
// Property accessors
@Id @GeneratedValue
@Column(name=”USER_ID”, unique=true, nullable=false)
public Integer getUserId() {
return this.userId;
}
public void setUserId(Integer userId) {
this.userId = userId;
}
@Column(name=”USER_NAME”, nullable=false, length=50)
public String getUserName() {
return this.userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
@Column(name=”PASSWORD”, nullable=false, length=20)
public String getPassword() {
return this.password;
}
public void setPassword(String password) {
this.password = password;
}
@Column(name=”CONTACT_NAME”, nullable=false, length=50)
public String getContactName() {
return this.contactName;
}
public void setContactName(String contactName) {
this.contactName = contactName;
}
@Column(name=”MOBILE_PHONE”, nullable=false, length=50)
public String getMobilePhone() {
return this.mobilePhone;
}
public void setMobilePhone(String mobilePhone) {
this.mobilePhone = mobilePhone;
}
@Column(name=”OFFICE_PHONE”, length=50)
public String getOfficePhone() {
return this.officePhone;
}
public void setOfficePhone(String officePhone) {
this.officePhone = officePhone;
}
@Column(name=”ADDRESS”, nullable=false)
public String getAddress() {
return this.address;
}
public void setAddress(String address) {
this.address = address;
}
@Column(name=”EMAIL”)
public String getEmail() {
return this.email;
}
public void setEmail(String email) {
this.email = email;
}
@Column(name=”STATUS”, nullable=false, length=10)
public String getStatus() {
return this.status;
}
public void setStatus(String status) {
this.status = status;
}
@Column(name=”CREATE_DATE”, nullable=false, length=0)
public Timestamp getCreateDate() {
return this.createDate;
}
public void setCreateDate(Timestamp createDate) {
this.createDate = createDate;
}
UserDAO
package dao;
import java.util.ArrayList;
import pojo.Users;
public class UserDAO {
public static Boolean Login(Users u)throws Exception{
String sql=”from users where userName=? and password=?”;
Object[]params={u.getUserName(),u.getPassword()};
ArrayList list=BaseDAO.runSelect(sql, params);
if(list.size()>0){
return true;
}else{
return false;
}
}
}
}
20
String sql=”select * from users where userName=? and password=?”;
20